AdMob is a mobile advertising platform that allows developers to monetize their mobile apps by displaying ads to users. If you are a developer using the Unity game engine, you may be interested in adding AdMob to your project in order to generate revenue.
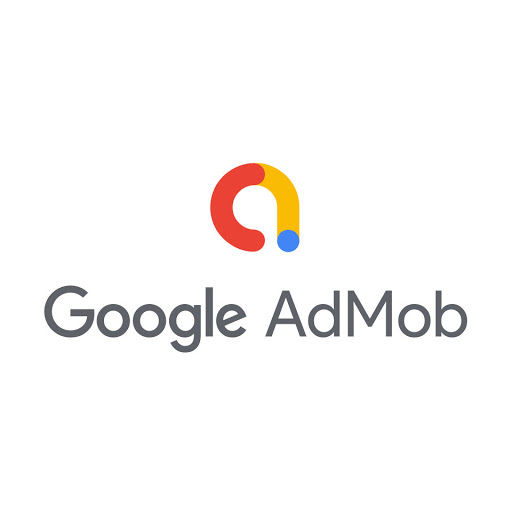
To add AdMob to your Unity project, you will need to follow a few steps. First, you will need to sign up for a Google AdMob account and create a new ad unit. This will give you a unique identifier for the ad unit, which you will need to include in your Unity project.
Next, you will need to download and install the Google Mobile Ads Unity plugin. This plugin provides the necessary libraries and assets to integrate AdMob into your Unity project. Once the plugin is installed, you will need to import it into your Unity project by going to "Assets > Import Package > Custom Package" and selecting the plugin file.
After importing the plugin, you will need to initialize the AdMob SDK by adding the following code to your project:
using GoogleMobileAds.Api;
void Start() {
// Initialize the Google Mobile Ads SDK.
MobileAds.Initialize(appId);
}
Replace "appId" with the unique identifier for your AdMob app, which you can find in the AdMob dashboard. This initialization step must be performed before you can start showing ads in your app.
Once the AdMob SDK is initialized, you can create an ad banner and display it in your app by using the following code:
using GoogleMobileAds.Api;
BannerView bannerView;
void Start() {
string adUnitId = "ca-app-pub-3940256099942544/6300978111";
// Create a banner view and add it to the view hierarchy.
bannerView = new BannerView(adUnitId, AdSize.Banner, AdPosition.Bottom); AdRequest request = new AdRequest.Builder().Build(); bannerView.LoadAd(request);
}
Replace "adUnitId" with the unique identifier for your ad unit, which you can find in the AdMob dashboard. The AdSize and AdPosition parameters specify the size and position of the ad banner on the screen.
In addition to banner ads, you can also display interstitial ads and rewarded ads in your Unity app. Interstitial ads are full-screen ads that are displayed at natural transition points in your app, such as between levels or when the app is launched. Rewarded ads are similar to interstitial ads, but they offer users a reward for watching the ad, such as in-game currency or items.
To display an interstitial ad, you can use the following code:
using GoogleMobileAds.Api; InterstitialAd interstitial;
void Start() {
string adUnitId = "ca-app-pub-3940256099942544/1033173712";
// Create an interstitial ad and add it to the view hierarchy. interstitial = new InterstitialAd(adUnitId);
AdRequest request = new AdRequest.Builder().Build(); interstitial.LoadAd(request);
}
voidShowInterstitial() {
if (interstitial.IsLoaded()) {
interstitial.Show(); }
}
Comments